An array is a container that stores and organizes items sequentially.
They are an ordered collection of elements and each value belongs to the same data type.
Indexing of the array start from 0 to n-1.
Similarities and Differences between Lists and Arrays
Similarities
Both lists and array are containers that stores and organizes items sequentially.
Lists and dynamic arrays are mutable and not fixed in size. Items can be added and removed, making them very flexible to work with.
Differences
Lists store items that are of various data types. This means that we can store integers, floats, strings, etc. all in one list.
Arrays store only items that are of the same single data type. This means that we can only store integers, or only strings, or only any other data type in arrays.
Lists are built in data structures in Python.
Arrays are not a built-in data structure, and therefore need to be imported via the array module
in order to be used.
Creating an Array
The first step to create array in Python is to import the array module
.
After importing, we will define the array using the syntax array(data_type, value_list)
. The data type and element list is specified in its arguments.
import array as arr
a = arr.array('i',[1,7,9])
# printing elements of the array
for element in a:
print(element)
Time Complexity: O(1)
Space Complexity: O(n)
Below is the typecode table that we can use to for the data type that needs to be specified in the argument.
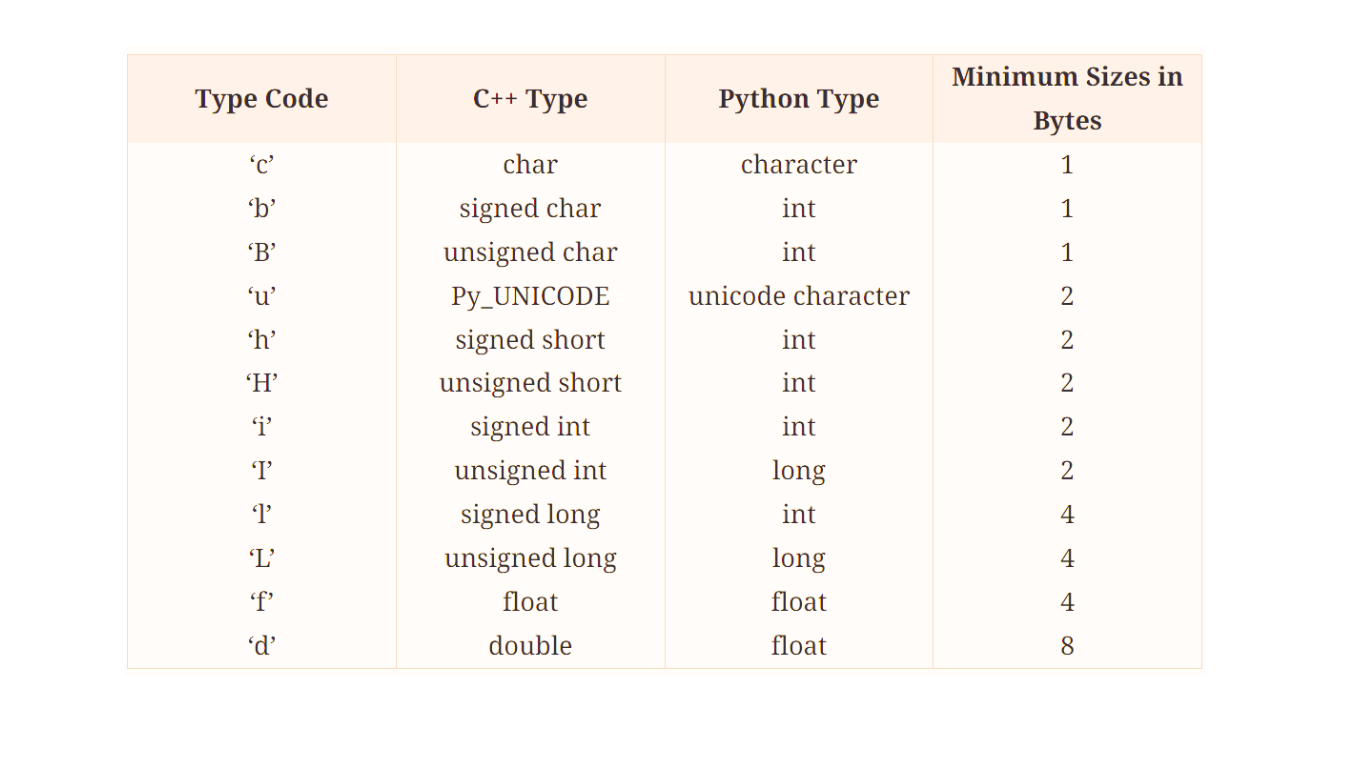
Getting Length of an Array
To get the number of elements in an array, we can use the built-in len() method
import array as arr
a = arr.array('i',[1,7,9])
# printing length of the array
print(len(a)) #3
Access and Search Elements in an Array
To access the elements of the array, we need to specify the index, to get the element at that index. The Time Complexity: O(1) and Auxiliary Space: O(1).
import array as arr
a = arr.array('i',[1,7,9])
print(a[0]) # 1
print(a[1]) # 7
print(a[2]) # 9
To search for the index of an element in the array, we will use the index(). The Time Complexity: O(n) and Auxiliary Space: O(1).
import array as arr
a = arr.array('i',[1,7,9])
print(a.index(9)) # 2
Note- To know more about how arrays work, check this post.
Slicing of an Array
To access a specific range of values inside the array, we use the slicing operator :
.
We specify the start index and the end index, separated by a colon. The end index element is not included.
import array as arr
a = arr.array('i',[1,7,9,10,7,5])
print(a[2:4]) # array('i', [9, 10])
By leaving out the start index, we can get the characters from the start to the position specified (not included in the output).
import array as arr
a = arr.array('i',[1,7,9,10,7,5])
print(a[:4]) # array('i', [1, 7, 9, 10])
By leaving out the end index, we can get the characters from the specified position , to all the way till the end.
import array as arr
a = arr.array('i',[1,7,9,10,7,5])
print(a[2:]) # array('i', [9, 10, 7, 5])
Change the value at specified index in an Array
We can change the value at specific index by specifying the index and assigning it a new value.
import array as arr
a = arr.array('i',[1,7,9,10,7,5])
a[0] = 4
print(a) # array('i', [4, 7, 9, 10, 7, 5])
Adding Elements to an Array
There are two ways to add element to an array
1) insert(index, element)
– used to add a new element at any given index of the array. The index and the element is specified in its arguments. Since we have to move other elements in the array to insert, the Time Complexity: O(n) and Space: O(1).
2) append()
– used to add a new element at the end of the array. Since we are adding at the end and not moving other elements in the array, so Time Complexity: O(1) and Space: O(1).
import array as arr
a = arr.array('i',[1,7,9])
a.insert(1,3) # inserting value 3 at index 1
print(a) # array('i', [1, 3, 7, 9])
a.append(8) # appending value 8 at the end
print(a) # array('i', [1, 3, 7, 9, 8])
Removing Elements from the Array
There are two ways to remove elements from an array
1) remove(value)
– only the first occurrence of the value passed in the argument is removed.
import array as arr
a = arr.array('i',[1,7,9,10,7,5])
a.remove(7) # removes only first occurence of 7
print(a) # array('i', [1, 9, 10, 7, 5])
2) pop()
– by default remove the last element of the array. To remove element from a specific position in the array, the index of the element is passed as an argument.
import array as arr
a = arr.array('i',[1,7,9,10,7,5])
a.pop() # pops last element
print(a) # array('i', [1, 7, 9, 10, 7])
a.pop(2) # pops element at index 2
print(a) # array('i', [1, 7, 10, 7])
The Time Complexity for both will be O(1) if we are removing elements at the end of the array and O(n) if we are removing elements at the any other position. The Space Complexity will be O(1).